1. 시험환경
˙ 구글 서치 콘솔
˙ 소유 도메인
2. 목적
˙ 구글 서치 콘솔에 도메인을 등록하여 소유권 확인하는 절차를 알아보자.
˙ 구글 검색노출을 위해 가장 먼저 진행되어야 하는 단계이다.
3. 적용
① 구글 서치 콘솔 사이트에 접속한다.
- URL : https://search.google.com/search-console/about?hl=ko
Google Search Console
Search Console 도구와 보고서를 사용하면 사이트의 검색 트래픽 및 실적을 측정하고, 문제를 해결하며, Google 검색결과에서 사이트가 돋보이게 할 수 있습니다.
search.google.com
② "시작하기" 버튼 클릭 후 로그인한다.
![]() |
![]() |
③ 좌측상단 "속성 검색"을 클릭하고 "+ 속성 추가"를 클릭한다.
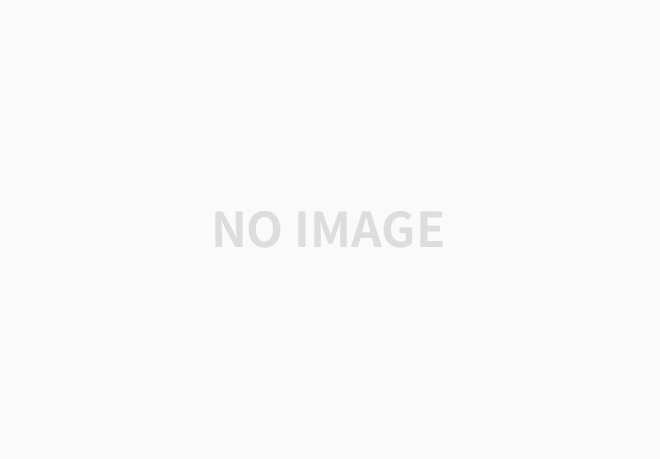
④ 등록할 URL을 입력한다.
- 도메인 : TXT 값을 DNS 사이트(AWS Route 53 등)에서 설정하며 example.com의 모든 서브 도메인에 적용된다.
- URL 접두어 : 설정 파일을 사이트마다 업로드하여 www.example.com와 와 같이 각각의 서브 도메인 마다 적용한다.
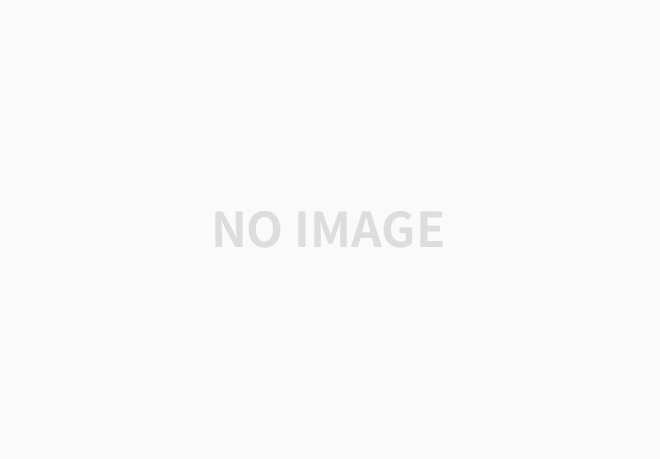
⑤ 태그를 복사하여 main 페이지에 추가한다.
- 도메인 선택 경우 : 제공된 TXT 값을 복사하여 DNS의 TXT 레코드에 설정한다.
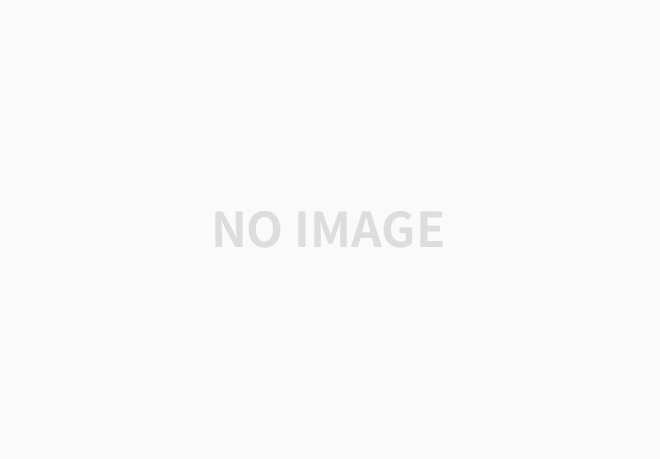
- URL 접두어 선택 경우 : 제공 파일을 다운로드하여 Hosting 사이트의 root 경로에 업로드한다.
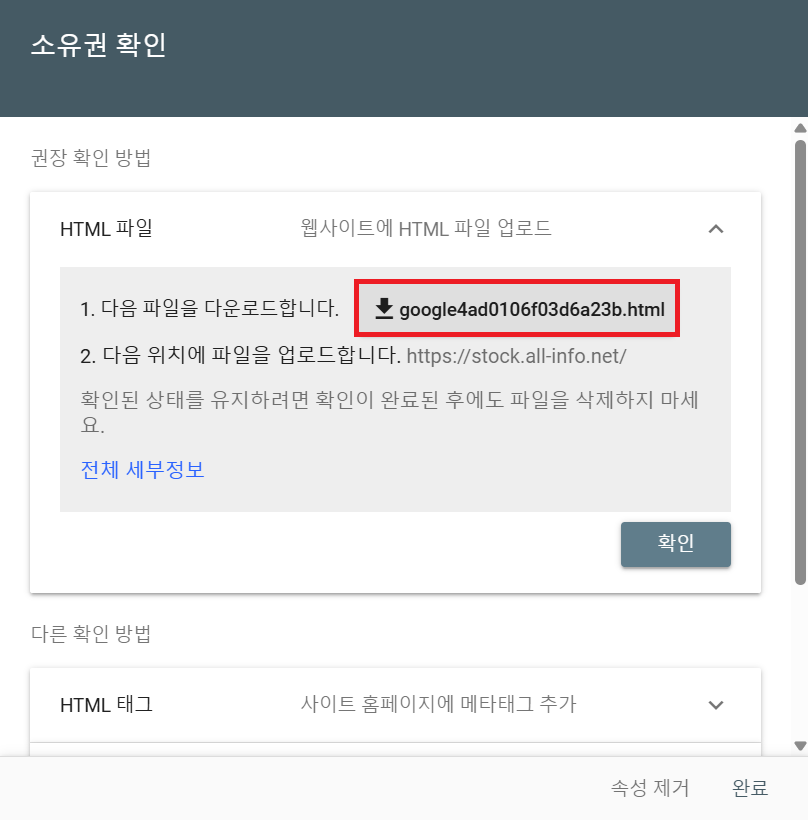
⑥ DNS(도메인 TXT 설정) 또는 Hosting 사이트(파일업로드)에서 설정 후 소유권이 확인되도록 한다.
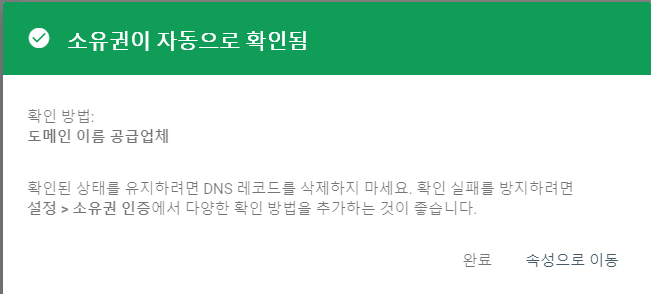
'웹 사이트' 카테고리의 다른 글
npm을 이용한 next.js 프로젝트 생성 (0) | 2025.04.06 |
---|---|
[워드프레스] 관리자 비밀번호 변경 (7) | 2024.06.27 |
Docker 기반 워드프레스 초기 설치 (1) | 2024.06.17 |
쿠키(Cookie)와 세션(Session) (0) | 2024.01.25 |
가비아(Gabia)에서 구매한 도메인(URL)을 EC2에 연결하기 (0) | 2023.07.03 |